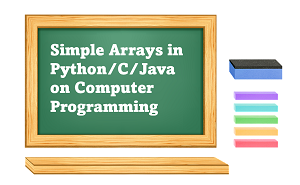
Arrays in Programming Tutorial
#include <stdio.h>
main() {
int number1;
int number2;
int number3;
int number4;
int number5;
number1 = 10;
number2 = 20;
number3 = 30;
number4 = 40;
number5 = 50;
printf( "number1: %d\n", number1);
printf( "number2: %d\n", number2);
printf( "number3: %d\n", number3);
printf( "number4: %d\n", number4);
printf( "number5: %d\n", number5);
}
It was straightforward, on the grounds that we needed to store only five-number numbers. Presently how about we expect we need to store 5000 whole numbers. Is it safe to say that we will utilize 5000 factors?
To deal with such circumstances, practically all the programming dialects give an idea called an exhibit. An exhibit is an information structure, which can store a fixed-size assortment of components of a similar information type. An exhibit is utilized to store an assortment of information, however, it is in many cases more valuable to consider a cluster of an assortment of factors of a similar sort.
Rather than announcing individual factors, for example, number1, number2, ..., number99, you simply pronounce one exhibit variable number of number kind and use number1[0], number1[1], and ..., number1[99] to address individual factors. Here, 0, 1, 2, .....99 are record related with var variable, and they are being utilized to address individual components accessible in the cluster.
All clusters comprise bordering memory areas. The least location relates to the principal component and the most elevated address to the last component.
Make Arrays
To make an exhibit variable in C, a developer determines the sort of the components and the number of components to be put away in that exhibit. Given beneath is a basic linguistic structure to make an exhibit in C programming.
type arrayName [ arraySize ];
This is known as a solitary layered cluster. The array size should be a whole number steady more noteworthy than nothing and type can be any substantial C information type. For instance, presently to proclaim a 10-component exhibit called number of type int, utilize this assertion.
int number[10];
Here, the number is a variable exhibit, which is adequate to hold up to 10 whole numbers.
Instating Arrays
You can introduce an exhibit in C it is possible that individuals or involving a solitary assertion as follows.
int number[5] = {10, 20, 30, 40, 50};
The quantity of values between supports { } can't be bigger than the quantity of components that we proclaim for the cluster between square sections [ ].
In the event that you discard the size of the cluster, an exhibit sufficiently enormous to hold the statement is made. Subsequently, in the event that you compose −
int number[] = {10, 20, 30, 40, 50};
You will make the very same cluster as you did in the past model. Following is a guide to dole out a solitary component of the exhibit −
number[4] = 50;
The above assertion doles out component number fifth in the cluster with a worth of 50. All clusters have 0 as the record of their most memorable component which is likewise called the base file, and the last record of an exhibit will be the absolute size of the cluster less 1. The accompanying picture shows the pictorial portrayal of the cluster we talked about above −
Getting to Array Elements
A component is gotten to by ordering the exhibit name. This is finished by setting the list of the component inside square sections after the name of the exhibit. For instance −
int var = number[9];
The above assertion will take the tenth component from the exhibit and allot the worth to the var variable. The accompanying model purposes every one of the previously mentioned three ideas viz. creation, task, and getting to clusters.
#include <stdio.h>
int main () {
int number[10]; /* number is an array of 10 integers */
int i = 0;
/* Initialize elements of array n to 0 */
while( i < 10 ) {
/* Set element at location i to i + 100 */
number[ i ] = i + 100;
i = i + 1;
}
/* Output each array element's value */
i = 0;
while( i < 10 ) {
printf("number[%d] = %d\n", i, number[i] );
i = i + 1;
}
return 0;
}
When the above code is compiled and executed, it produces the following result.
number[0] = 100
number[1] = 101
number[2] = 102
number[3] = 103
number[4] = 104
number[5] = 105
number[6] = 106
number[7] = 107
number[8] = 108
number[9] = 109
Arrays in Java
Following is the same program written in Java. Java upholds exhibits, however there is a little contrast in the manner they are made in Java utilizing the new administrator.
You can attempt to execute the accompanying system to see the result, which should be indistinguishable from the outcome produced by the above C model.
public class DemoJava {
public static void main(String []args) {
int[] number = new int[10];
int i = 0;
while( i < 10 ) {
number[ i ] = i + 100;
i = i + 1;
}
i = 0;
while( i < 10 ) {
System.out.format( "number[%d] = %d\n", i, number[i] );
i = i + 1;
}
}
}
When the above program is executed, it produces the following result.
number[0] = 100
number[1] = 101
number[2] = 102
number[3] = 103
number[4] = 104
number[5] = 105
number[6] = 106
number[7] = 107
number[8] = 108
number[9] = 109
Arrays (Lists) in Python
Python does not have a concept of Array, instead Python provides another data structure called list, which provides similar functionality as arrays in any other language.
Following is the equivalent program written in Python:
# Following defines an empty list.
number = []
i = 0
while i < 10:
# Appending elements in the list
number.append(i + 100)
i = i + 1
i = 0
while i < 10:
# Accessing elements from the list
print "number[", i, "] = ", number[ i ]
i = i + 1
When the above program is executed, it produces the following result −
number[ 0 ] = 100
number[ 1 ] = 101
number[ 2 ] = 102
number[ 3 ] = 103
number[ 4 ] = 104
number[ 5 ] = 105
number[ 6 ] = 106
number[ 7 ] = 107
number[ 8 ] = 108
number[ 9 ] = 109