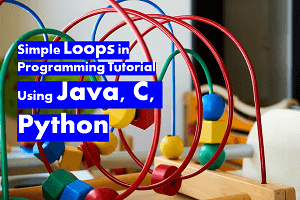
The Loops in Programming Tutorials
Let's consider when you want to print Hello World! 5x. A simple C program example.
#include <stdio.h>
main() {
printf( "Hello, World!\n");
printf( "Hello, World!\n");
printf( "Hello, World!\n");
printf( "Hello, World!\n");
printf( "Hello, World!\n");
}
Below is the result.
Hello World!
Hello World!
Hello World!
Hello World!
Hello World!
It was basic, but, let's consider another thing when you want to code Hello World! A many times. We can certainly not write printf() statements a thousand times. Almost all computer programming languages provide loops, which needs to action statements. All high-level computer programming languages give various forms of loops, which can be used to start one or more statements repeatedly.
Let's write an example above C program with the while loop, we will explain how loop works
#include <stdio.h>
main() {
int i = 0;
while ( i < 5 ) {
printf( "Hello, World!\n");
i = i + 1;
}
}
Below is the result.
Hello World!
Hello World!
Hello World!
Hello World!
Hello World!
The above example makes use of a while loop, which is being used to start a set of statements enclosed within {....}. Here, the computer first verifies whether the given condition, i.e., variable "a" is less than 5 or not and if it finds the condition is true, then the loop body is entered to start the given statements. Here, we have the following two statements in the loop body.
- printf() function, which prints Hello World!
- i = i + 1, which is used to increase the value of variable i
After starting all the statements given in the loop body, the computer goes back to while( i < 5) and the given condition, (i < 5), is checked again, and the loop is executed again if the condition holds true. This process repeats till the given condition remains true, which means variable "a" has a value less than 5.
To conclude, a loop statement allows us to execute a statement or group of statements multiple times. Given below is the general form of a loop statement in most of the programming languages.
This tutorial has been designed to present programming's basic concepts to non-programmers, so let's discuss the two most important loops available in C programming language. Once you are clear about these two loops, then you can pick up a C programming tutorial or a reference book and check other loops available in C and the way they work.
The while Loop
A while loop available in C Programming language has the following syntax.
while ( condition ) {
/*... while loop ...*/
}
The following important points are to be noted about a while loop.
- A while loop starts with a keyword while followed by a condition enclosed in ( ).
- Further to the while() statement, you will have the body of the loop enclosed in curly braces {...}.
- A while loop body can have one or more lines of source code to be executed repeatedly.
- If the body of a while loop has just one line, then it's optional to use curly braces {...}.
- A while loop keeps executing its body till a given condition holds true. As soon as the condition becomes false, the while loop comes out and continues executing from the immediate next statement after the while loop body.
- A condition is usually a relational statement, which is evaluated to either true or false. A value equal to zero is treated as false, and any non-zero value works like true.
The Do While Loop
Sometimes while loop a given condition before it executes any assertions given in the body part. C programming gives one more type of looping, called do...while, that permits to execute of a circle body prior to really looking at a given condition. It has the accompanying syntax.
do {
/*....do...while loop body ....*/
} while ( condition );
The above code can be represented in the form of a flow diagram as shown below.
If you write the above example using do...while loop, then Hello World will produce the same result.
#include <stdio.h>
main() {
int i = 0;
do {
printf( "Hello World!\n");
i = i + 1;
}
while ( i < 5 );
}
Below is the result
Hello World!
Hello World!
Hello World!
Hello World!
Hello World!
The break statement
When the break statement is encountered a loop, it is urgently stop and the program resumes at the next statement. The example for a break statement in C syntax below.
break;
A break statement can be addressed as a stream graph, as displayed underneath.
Following is a variation of the above program, yet it will emerge after printing Hello World! Just multiple times.
#include <stdio.h>
main() {
int i = 0;
do {
printf( "Hello, World!\n");
i = i + 1;
if( i == 3 ) {
break;
}
}
while ( i < 5 );
}
Below is the result.
Hello World!
Hello World!
Hello World!
The continue statement
To proceed with explanation in C programming language works to some degree like the break proclamation. Rather than compelling end, proceed with powers the following emphasis of the circle to occur, skirting any in the middle between. The grammar for proceed with explanation in C is as per the following.
continue;
A continue statement can be represented in the form of a flow diagram, as shown below.
The following is a variant of the above program, but it will skip printing when the variable has a value equal to 3.
#include <stdio.h>
main() {
int i = 0;
do {
if( i == 3 ) {
i = i + 1;
continue;
}
printf( "Hello World!\n");
i = i + 1;
}
while ( i < 5 );
}
Below is the result.
Hello World!
Hello World!
Hello World!
Hello World!
Loops in Java
Following is the same program written in Java that also upholds while and do...while circles. The accompanying system prints Hello World! Multiple times, as we did on account of C Programming −
You can attempt to execute the accompanying project to see the result, which should be indistinguishable from the outcome created by the above model.
public class DemoJava {
public static void main(String []args) {
int i = 0;
while ( i < 5 ) {
System.out.println("Hello World!");
i = i + 1;
}
}
}
The break and continue statements in Java programming work quite the same way as they work in C programming.
Loops in Python
Following is the same program written in Python. Python also upholds while and do...while circles. The accompanying project prints Hello World! Multiple times, as we did if there should be an occurrence of C Programming. Here you should take note of that Python doesn't utilize wavy supports for the circle body, rather it essentially recognizes the body of the circle utilizing space of the assertions. You can attempt to execute the accompanying project to see the result.
To show the distinction, we have utilized another print explanation, which will be executed when the circle will be finished.
i = 0
while (i < 5):
print "Hello World!"
i = i + 1
print "Loop ends"
Below is the result.
Hello World!
Hello World!
Hello World!
Hello World!
Hello World!
Loop ends
The break and proceed with articulations in Python work a remarkable same way as they do in C programming.